Hexadecimals are hard
Still working through Daniel Weller and Sharat Chikkerur's course 6.087 on MIT OCW [1] and I struggled with hexadecimals. I wanted to make some notes on how they work for later reference.
The hexadecimal system is base 16. It uses the numbers 0 - 9 and letters A - F to denote the value for a decimal place [2]. Thanks to Wikipedia for a table showing the conversions between hex, binary (base 2), octal (base 8) and decimal (base 10):
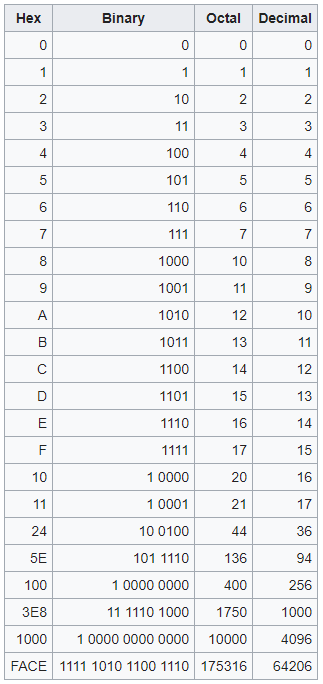
Source: https://simple.wikipedia.org/wiki/Hexadecimal_numeral_system
Notice that a single hexadecimal digit is equivalent to 4 bits; 2 hexadecimal digits are equivalent to 1 byte. In C, hexadecimal numbers can be initialized as integers and then denoted with 0x at the beginning. For example:
int value = 0x00FF ;
You can show a value in hexadecimal using the printf function like this:
printf( "\n %0X \n", value ) ;
where 0 left-pads the number with zeroes where spacing is specified and X denotes an unsigned hexadecimal integer in capital letters [3].
I also played with bitwise operators on this p-set. The key bitwise operators are [4]:
& - bitwise AND
| - bitwise OR
~ - bitwise NOT
^ - bitwise XOR
>> - right shift
<< - left shift
The truth table for the operators is shown below:
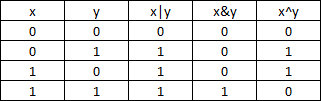
Source: https://fresh2refresh.com/c-programming/c-operators-expressions/c-bit-wise-operators/
Notice that the difference between OR and XOR (also called "exclusive OR") is that if x and y are both 1, the value of OR is 1, but because XOR is only true if only one of the inputs are true, then when x and y are both 1, the value of XOR is 0 [5]. Note that you can denote how many bits to shift left or right like this:
value >> 4 ;
This expression right shifts value by 4 bits.
Sources:
[1] Weller, D and Chikkerur, S, MIT OCW 6.087 Practical Programming in C, https://ocw.mit.edu/courses/electrical-engineering-and-computer-science/6-087-practical-programming-in-c-january-iap-2010/index.htm.
[2] https://simple.wikipedia.org/wiki/Hexadecimal_numeral_system
[3] https://www.tutorialspoint.com/c_standard_library/c_function_printf.htm
[4] https://fresh2refresh.com/c-programming/c-operators-expressions/c-bit-wise-operators/
[5] https://en.wikipedia.org/wiki/Exclusive_or